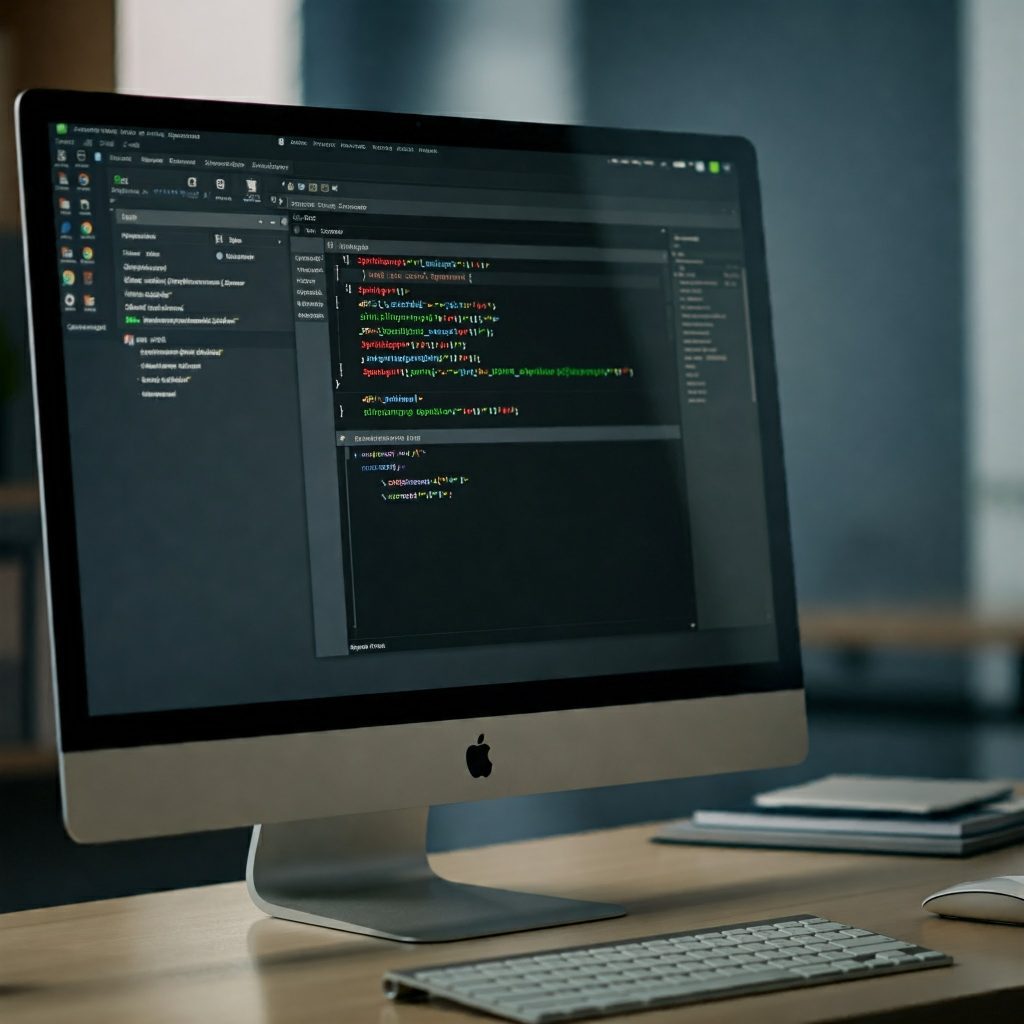
Working with Profiles in Spring Boot – Environment-Specific Configuration
In the world of modern application development, the ability to adapt to different environments is crucial. Whether you’re developing locally, testing in a staging environment, or deploying to production, your application needs to behave appropriately in each context. This is where Spring Boot profiles come into play, offering a powerful mechanism for managing environment-specific configurations. By leveraging profiles, developers can streamline their workflow, enhance application flexibility, and ensure smooth transitions between various deployment stages. This blog post delves into the intricacies of working with profiles in Spring Boot, providing a comprehensive guide to implementing environment-specific configurations effectively.
Understanding Spring Boot Profiles
What are Spring Boot Profiles?
Spring Boot profiles are a core feature of the Spring Framework that allows developers to segregate parts of their application configuration and make it available only in certain environments. Profiles provide a way to define beans that should only be created in specific contexts, allowing for greater flexibility and modularity in application design. They enable developers to activate different configurations based on the current environment, such as development, testing, or production, without changing the application code.
Why Use Profiles?
The use of profiles in Spring Boot applications offers several compelling advantages:
- Environment-specific configurations: Profiles allow you to define different sets of configuration properties for various environments, ensuring that your application behaves correctly whether it’s running on a developer’s local machine or in a production server.
- Simplified deployment process: By encapsulating environment-specific settings within profiles, you can streamline the deployment process and reduce the risk of configuration errors when moving between environments.
- Enhanced testing capabilities: Profiles enable you to create specific configurations for testing scenarios, making it easier to isolate and verify different aspects of your application’s behavior.
- Improved code organization: By separating configuration concerns based on environments, profiles contribute to cleaner, more maintainable code structures.
- Dynamic behavior adjustment: Profiles allow your application to adapt its behavior at runtime based on the active profile, providing greater flexibility in how your application operates in different contexts.
Creating and Configuring Profiles
Defining Profiles
In Spring Boot, profiles can be defined in several ways. The most common approach is to create separate property files for each environment, following a naming convention that includes the profile name. For example:
application.properties
: Default propertiesapplication-dev.properties
: Development-specific propertiesapplication-prod.properties
: Production-specific properties
Here’s an example of how these files might be structured:
src/
main/
resources/
application.properties
application-dev.properties
application-prod.properties
Configuring Profile-Specific Properties
Within each profile-specific property file, you can define properties that are unique to that environment. For instance:
# application-dev.properties
server.port=8080
logging.level.root=DEBUG
database.url=jdbc:mysql://localhost:3306/devdb
# application-prod.properties
server.port=80
logging.level.root=INFO
database.url=jdbc:mysql://production-server:3306/proddb
In this example, we’ve defined different server ports, logging levels, and database URLs for the development and production environments.
Using @Profile Annotation
Spring Boot also allows you to use the @Profile
annotation to specify that a component or configuration should only be active when a specific profile is active. This is particularly useful for beans that should only exist in certain environments:
@Configuration
@Profile("dev")
public class DevConfig {
@Bean
public DataSource dataSource() {
// Development-specific DataSource configuration
}
}
@Configuration
@Profile("prod")
public class ProdConfig {
@Bean
public DataSource dataSource() {
// Production-specific DataSource configuration
}
}
In this example, the appropriate DataSource
bean will be created based on the active profile.
Activating Profiles
Setting the Active Profile
There are several ways to activate a specific profile in a Spring Boot application:
- Using the
spring.profiles.active
property:
- In
application.properties
:spring.profiles.active=dev
- As a command-line argument:
java -jar myapp.jar --spring.profiles.active=prod
- As an environment variable:
export SPRING_PROFILES_ACTIVE=prod
- Programmatically using
SpringApplication
:
public static void main(String[] args) {
SpringApplication app = new SpringApplication(MyApplication.class);
app.setAdditionalProfiles("dev");
app.run(args);
}
- Using the
@ActiveProfiles
annotation in test classes:
@RunWith(SpringRunner.class)
@SpringBootTest
@ActiveProfiles("test")
public class MyApplicationTests {
// Test methods
}
Profile Hierarchies and Inheritance
Spring Boot supports profile hierarchies, allowing you to create more complex profile configurations. You can activate multiple profiles simultaneously, and Spring will merge the properties from all active profiles. If there are conflicts, the last loaded profile takes precedence.
For example, you might have a base “default” profile, with more specific profiles layered on top:
application.properties (default)
application-db.properties (database-specific)
application-dev.properties (development environment)
You could then activate these profiles together:
spring.profiles.active=db,dev
This approach allows for more modular and reusable profile configurations.
Best Practices for Working with Profiles
Organizing Profile-Specific Configurations
When working with profiles in Spring Boot, it’s essential to maintain a clear and organized structure for your configuration files. Here are some best practices to consider:
- Use a consistent naming convention for profile-specific property files.
- Group related properties together within each file for better readability.
- Consider using YAML files instead of properties files for more complex configurations.
- Document the purpose and usage of each profile in comments or separate documentation.
Handling Sensitive Information
When dealing with sensitive information such as API keys or database credentials, it’s crucial to handle them securely:
- Never commit sensitive information to version control.
- Use environment variables or external configuration servers for sensitive data.
- Consider using Spring Cloud Config for centralized configuration management.
- Implement encryption for sensitive properties using tools like Jasypt.
Testing with Profiles
Profiles can greatly enhance your testing strategy:
- Create separate profiles for different testing scenarios (e.g., integration tests, performance tests).
- Use the
@ActiveProfiles
annotation to activate specific profiles in test classes. - Implement profile-specific mock beans for external services or resources.
Advanced Profile Techniques
Conditional Bean Creation
Spring Boot’s @Conditional
annotations can be used in conjunction with profiles for more fine-grained control over bean creation:
@Configuration
public class DatabaseConfig {
@Bean
@Profile("dev")
@ConditionalOnProperty(name = "use.in-memory.database", havingValue = "true")
public DataSource inMemoryDataSource() {
// Configure and return an in-memory database
}
@Bean
@Profile("prod")
@ConditionalOnProperty(name = "use.cloud.database", havingValue = "true")
public DataSource cloudDataSource() {
// Configure and return a cloud database connection
}
}
This example demonstrates how you can create beans conditionally based on both the active profile and specific property values.
Profile Groups
Spring Boot 2.4 introduced the concept of profile groups, allowing you to activate multiple profiles with a single declaration:
spring.profiles.group.production=prod,audit,ha
spring.profiles.group.development=dev,debug
With this configuration, activating the “production” profile group would automatically activate the “prod”, “audit”, and “ha” profiles.
Dynamic Profile Selection
In some cases, you might want to select profiles dynamically based on runtime conditions. You can achieve this by implementing a custom EnvironmentPostProcessor
:
public class DynamicProfileSelector implements EnvironmentPostProcessor {
@Override
public void postProcessEnvironment(ConfigurableEnvironment environment, SpringApplication application) {
if (someCondition()) {
environment.addActiveProfile("profile1");
} else {
environment.addActiveProfile("profile2");
}
}
private boolean someCondition() {
// Implement your condition logic here
}
}
Remember to register your custom EnvironmentPostProcessor
in META-INF/spring.factories
:
org.springframework.boot.env.EnvironmentPostProcessor=com.example.DynamicProfileSelector
Implementing Profile-Specific Features
Database Configurations
One common use case for profiles is managing different database configurations. Here’s an example of how you might set up profile-specific database properties:
# application-dev.properties
spring.datasource.url=jdbc:h2:mem:devdb
spring.datasource.driver-class-name=org.h2.Driver
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
# application-prod.properties
spring.datasource.url=jdbc:mysql://prodserver:3306/proddb
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.database-platform=org.hibernate.dialect.MySQL8Dialect
Logging Configurations
Adjusting logging levels based on the environment is another common profile-based configuration:
# application-dev.properties
logging.level.root=DEBUG
logging.level.org.springframework.web=DEBUG
logging.level.org.hibernate=DEBUG
# application-prod.properties
logging.level.root=WARN
logging.level.org.springframework.web=ERROR
logging.level.org.hibernate=ERROR
External Service Configurations
Profiles can also be used to manage connections to external services:
# application-dev.properties
external.service.url=http://dev-api.example.com
external.service.api-key=dev-api-key
# application-prod.properties
external.service.url=http://api.example.com
external.service.api-key=${PROD_API_KEY}
In this example, we’re using an environment variable (${PROD_API_KEY}
) for the production API key to enhance security.
Troubleshooting Profile Issues
When working with profiles, you may encounter some common issues. Here are some troubleshooting tips:
- Profile not activating: Ensure that you’ve correctly set the active profile using one of the methods described earlier. You can verify the active profiles by logging
environment.getActiveProfiles()
at application startup. - Properties not being applied: Check the loading order of your property files. Remember that properties in
application.properties
are overridden by profile-specific properties. - Conflicts between profiles: If you’re activating multiple profiles, be aware of the order in which they’re loaded. The last loaded profile takes precedence for conflicting properties.
- Bean definition conflicts: When using
@Profile
on beans, ensure that you don’t have conflicting bean definitions across different profiles. - Profile-specific beans not being created: Verify that the profile name in your
@Profile
annotation matches exactly with the activated profile name.
Conclusion
Working with profiles in Spring Boot provides a powerful mechanism for managing environment-specific configurations. By leveraging profiles effectively, developers can create more flexible, maintainable, and robust applications that adapt seamlessly to different deployment contexts. From simple property file configurations to advanced techniques like profile groups and dynamic profile selection, Spring Boot offers a rich set of tools for tailoring your application’s behavior to various environments.
As you implement profiles in your Spring Boot applications, remember to follow best practices for organization, security, and testing. Regularly review and refactor your profile configurations to ensure they remain clean, efficient, and aligned with your application’s evolving needs. With a solid understanding of Spring Boot profiles, you’ll be well-equipped to develop applications that perform optimally across all stages of the development lifecycle, from local development to production deployment.
Disclaimer: While every effort has been made to ensure the accuracy and reliability of the information presented in this blog post, it is provided for educational purposes only. The examples and techniques described may need to be adapted to fit specific use cases and environments. Readers are encouraged to consult official Spring Boot documentation and conduct thorough testing before implementing these concepts in production systems. If you notice any inaccuracies or have suggestions for improvement, please report them so we can correct them promptly.