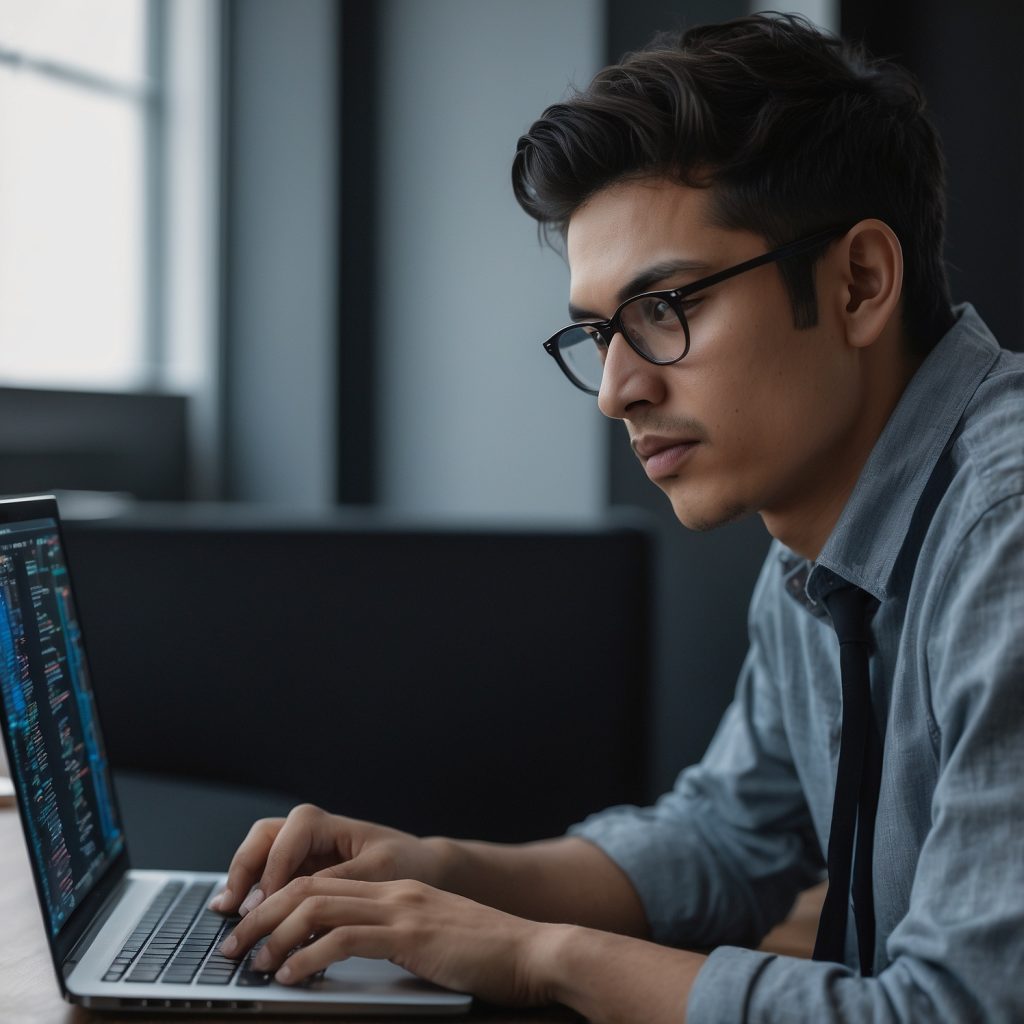
Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for any software development project. It ensures that your code is understandable, easy to modify, and less prone to bugs. In this blog, we’ll explore various practices and techniques to help you write code that stands the test of time.
Why Clean Code Matters
Readability and Understanding
Clean code is easy to read and understand. This is crucial because, in a team setting, multiple developers will work on the same codebase. When your code is readable, it reduces the time spent trying to understand what the code does. Here’s a simple example of clean vs. messy code:
# Messy Code
def calc(a, b):
return a+b, a-b, a*b, a/b
# Clean Code
def calculate_operations(num1, num2):
sum_result = num1 + num2
difference = num1 - num2
product = num1 * num2
quotient = num1 / num2
return sum_result, difference, product, quotient
The clean code example uses meaningful function and variable names, making it clear what each part of the code does.
Ease of Maintenance
Maintaining a clean codebase is much simpler. When bugs arise or new features need to be added, having well-organized code helps developers quickly locate and fix issues without causing additional problems. Consider the following refactoring for clarity and maintenance:
# Initial implementation
def process(data):
for item in data:
if item.status == 'active':
print('Active:', item.name)
elif item.status == 'inactive':
print('Inactive:', item.name)
# Refactored for maintainability
def process_active(item):
print('Active:', item.name)
def process_inactive(item):
print('Inactive:', item.name)
def process(data):
for item in data:
if item.status == 'active':
process_active(item)
elif item.status == 'inactive':
process_inactive(item)
By breaking down the process into smaller functions, we make the code easier to read and maintain.
Principles of Writing Clean Code
Follow Coding Standards
Adhering to coding standards ensures consistency across the codebase. This includes naming conventions, code formatting, and file organization. For example, in Python, following the PEP 8 style guide is a common practice.
# PEP 8 compliant example
class Employee:
def __init__(self, first_name, last_name, salary):
self.first_name = first_name
self.last_name = last_name
self.salary = salary
def full_name(self):
return f"{self.first_name} {self.last_name}"
def annual_salary(self):
return self.salary * 12
Keep It Simple (KISS)
The KISS principle advocates for simplicity. Complex code can be difficult to understand and maintain. Strive for simple solutions that achieve the desired functionality without unnecessary complexity.
# Complex approach
def calculate_discount(price, discount):
if discount == 0:
return price
elif discount > 0 and discount < 100:
return price - (price * (discount / 100))
else:
return price
# Simplified approach
def calculate_discount(price, discount):
return price - (price * (discount / 100)) if discount > 0 and discount < 100 else price
Don’t Repeat Yourself (DRY)
The DRY principle emphasizes the importance of reducing repetition. Duplicate code can lead to inconsistencies and make maintenance harder. Use functions or classes to encapsulate reusable logic.
# Repetitive code
def calculate_area_rectangle(length, width):
return length * width
def calculate_area_square(side):
return side * side
# DRY approach
def calculate_area(shape, dimension1, dimension2=None):
if shape == 'rectangle':
return dimension1 * dimension2
elif shape == 'square':
return dimension1 * dimension1
Write Self-Documenting Code
Self-documenting code is written in a way that is easy to understand without requiring extensive comments. This involves using meaningful variable names, clear logic, and well-structured functions.
# Self-documenting code example
def find_user_by_id(users, user_id):
for user in users:
if user.id == user_id:
return user
return None
Test-Driven Development (TDD)
TDD is a practice where you write tests before writing the actual code. This approach ensures that your code meets the requirements and behaves as expected. Here’s a simple example using Python’s unittest framework:
import unittest
def add(a, b):
return a + b
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(1, 2), 3)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(0, 0), 0)
if __name__ == '__main__':
unittest.main()
Writing Maintainable Code
Modular Design
Breaking down your code into smaller, independent modules makes it easier to manage. Each module should have a single responsibility, making it easier to update and test.
# Modular design example
class Database:
def connect(self):
pass
def query(self, sql):
pass
class UserRepository:
def __init__(self, database):
self.database = database
def get_user_by_id(self, user_id):
return self.database.query(f"SELECT * FROM users WHERE id = {user_id}")
# Main application
db = Database()
user_repo = UserRepository(db)
user = user_repo.get_user_by_id(1)
Consistent Naming Conventions
Use consistent naming conventions throughout your codebase. This includes variable names, function names, and class names. Consistency helps other developers understand your code more easily.
# Consistent naming example
def calculate_total_price(price, tax_rate):
return price + (price * tax_rate)
def is_valid_user(user):
return user.age >= 18
Code Reviews
Code reviews are an essential practice for maintaining code quality. They involve having other developers review your code to catch errors, suggest improvements, and ensure adherence to coding standards.
Documentation
While self-documenting code is ideal, comprehensive documentation is still necessary. Document your codebase, especially the parts that are complex or not immediately clear. Use docstrings in Python to provide inline documentation.
def add(a, b):
"""
Adds two numbers together.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
Refactoring
Regularly refactor your code to improve its structure and readability. Refactoring involves making small changes to the code without changing its functionality, aiming to improve the internal structure.
# Before refactoring
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
# After refactoring
def is_even(number):
return number % 2 == 0
def double(number):
return number * 2
def process_data(data):
return [double(item) for item in data if is_even(item)]
Sample Code and Scripts
Python Script for File Operations
Here’s an example of a clean and maintainable Python script for basic file operations:
import os
def read_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read()
except FileNotFoundError:
return "File not found."
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
return "Write successful."
def append_to_file(file_path, content):
with open(file_path, 'a') as file:
file.write(content)
return "Append successful."
def main():
file_path = 'example.txt'
print(write_file(file_path, "Hello, world!\n"))
print(append_to_file(file_path, "This is an appended line.\n"))
print(read_file(file_path))
if __name__ == "__main__":
main()
JavaScript Function for Array Manipulation
Below is an example of a clean and maintainable JavaScript function for manipulating arrays:
function filterAndMap(array, filterFn, mapFn) {
return array.filter(filterFn).map(mapFn);
}
const numbers = [1, 2, 3, 4, 5, 6];
const isEven = num => num % 2 === 0;
const double = num => num * 2;
const result = filterAndMap(numbers, isEven, double);
console.log(result); // Output: [4, 8, 12]
Writing clean and maintainable code is a skill that every developer should strive to master. By following principles such as adhering to coding standards, keeping your code simple, avoiding repetition, writing self-documenting code, and employing test-driven development, you can create code that is easy to understand, maintain, and extend. Additionally, using modular design, consistent naming conventions, regular code reviews, thorough documentation, and continuous refactoring will further enhance the maintainability of your codebase.
Remember, clean code is not just about making your life easier but also about being considerate of other developers who may work on your code in the future. By investing time and effort into writing clean and maintainable